Welcome to our third Blog, you will see information about the coding conventions followed, whom this application is meant for, prototype and UI of the MoneyTracker application and key changes
Coding Conventions
A coding standards document tells developers how they must write and present their code. Since the standard set is going to remain the same, its always a good practice to follow the coding standards so that its gonna make things easier to undersatand and more clear to the other developers working on the same application. Instead of each developer coding in their own preferred style, they will write all code to the standards outlined by the organisation or team. This makes sure that a large project is coded in a consistent style and parts are not written differently by different programmers. Not only does this solution make the code easier to understand, but also ensures that any developer who looks at the code will be able to get a clear picture on the structure of entire application.
Following are the coding conventions that we will be followed by our team:
1.1 Naming conventions :
The initial package name representing the domain name must be in lower case. For example, de.ovgu.janardhanast.name.login
Classes are always noun and are written in UpperCamelCase.
Example: SignInActivity.javaVariables always starts with lowercase letter and has mixed case,i.e. it follows camel case convention.
Example : Button btnLogin;The variables used to store UI components values satrts with the components followed by noun.
Example : EditText component used to enter UserName will be named as - EditText etUserName;
Radio button that holds income value is named as - RadioButton radiobtnIncome;-
Functions are always verb and named in accordance to what they do, and are written in lowercase
Every method and class will hold comments so as to what it does, hence allowing user to quickly understand the functionality
Constants are always written in Uppercase with underscore separating the words.
Example : int NUMBER_OF_CATEGORY=5;Resource IDs and names are written in lowercase_name.
1.2 Layout files :
Layout files should match the name of the Android components that they are intended for. Example : if we are creating a layout for the LoginActivity, the name of the layout file should be activity_login.xml.
1.3 Values files :
Resource files in the values folder should be plural,
Example : strings.xml, styles.xml, colors.xml, dimens.xml, attrs.xml
1.4 File import :
While importing a file, the import statement should have the complete file name prefixed by its package name.
Example : import com.example.sony.homeMain.MainActivity;
1.5 Exception handling :
All possible and expected exceptions are to be handled by using a try catch block with appropriate exceptions in the catch block
Example :
try {
// Protected code
}catch(ExceptionName e1) {
// Catch block
}
1.6 Space indentation :
4 space indents should be used for blocks.
Example :
if (switchState == true) {
//content
}
1.7 Brace style :
Braces go on the same line as the code. If the condition has multiple statements, it should be covered inside the braces.
Example :
If (isPinValid) {
Intent intent = new Intent(this, MainActivity.class );
startActivity(intent);
} else {
Intent intent = new Intent(this, LoginToHome.class);
startActivity(intent);
}
1.8 Logging :
Logging methods has to be used since we work as a team. We might not know whose file throws an error. If all these are captured in a log it will be quite useful for fellow developers.
Example :
public validatePin() {
Log.e(TAG, "Inside validate PIN method");
}
1.9 XML style rules :
ID of an XML element should have its asset type as prefix.
Example : button_login
If an XML element doesn't have any content, its a good practice to use self closing tags
Example :
< Spinner
android:id="@+id/txnCategory"
android:layout_width="220dp"
android:layout_height="50dp"/>
1.10 Annotations style :
Annotations should be written just above the method declaration and only one annotation per line
Example :
@override
public void onCreate(SQLiteDatabase db) {
SqliteHelper.onCreate(db);
}
1.10 Code format :
Code has to be formated using Ctrl+Alt+l once coding is completed. Before declaring a method one should always follow the practice of adding comments about what the method does.
Question is how do we make sure that all code adeheres to the coding conventions?
Coding standards are defined by discussing with the team, with all the good practices each one is aware of and it has been documented and shared, such that each developer follows the standard
Once coding is done and tested, code is reviewed by any one of the peer and it is guaranteed if coding conventions are followed and quality of code is maintained
Comment will be added to the related issue during code review phase, if the developer fails to meet any standard criterias that has been defined
To whom is our MoneyTracker Application more suitable?
Money Tracker is general purpose application suitable for every individual who is concerned to maintain or track the expenditure, none the less certain pre-requisites are, one willing to use the application has to be aware about usage of mobile phones, installation of android applications. This application can be better utilized by the pupils with age > 8 years, since it involves mathematically calculated results and representation of expenditure and savings interms of graph and Pie charts. This application is only focused for individuals who wish to track their day to day expenses, not for group of people or doesn't target large scale industrial expenditure maintainance
So considering the above factors we can define the two personas whom the application is more suitable is as follows:
1. Site Engineer
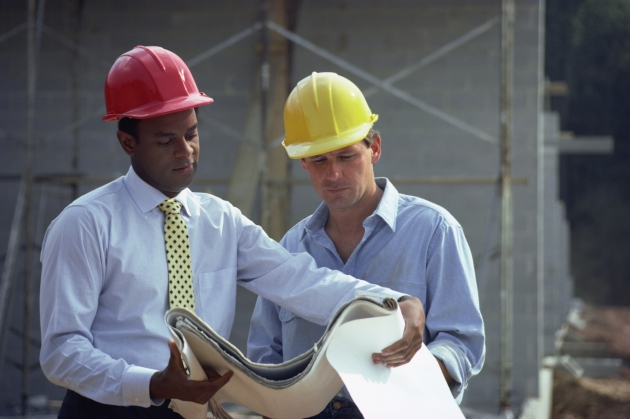
Persona |
Site Engineers |
||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|
Fictional name |
Sebastian Schroder |
||||||||||
Major responsibilities |
Inspection of sites and the status of construction elements |
||||||||||
Demographics |
30 years old, Site Engineer, German and Unmarried |
||||||||||
Goals and tasks |
As a site Engineer, he has to visit all the construction sites that he is responsible for. He |
||||||||||
Environment |
As an Engineer, He has to calculate various things regarding his construction sites and |
||||||||||
Quote |
Make life simpler with BIM app! |
2. Site Manager
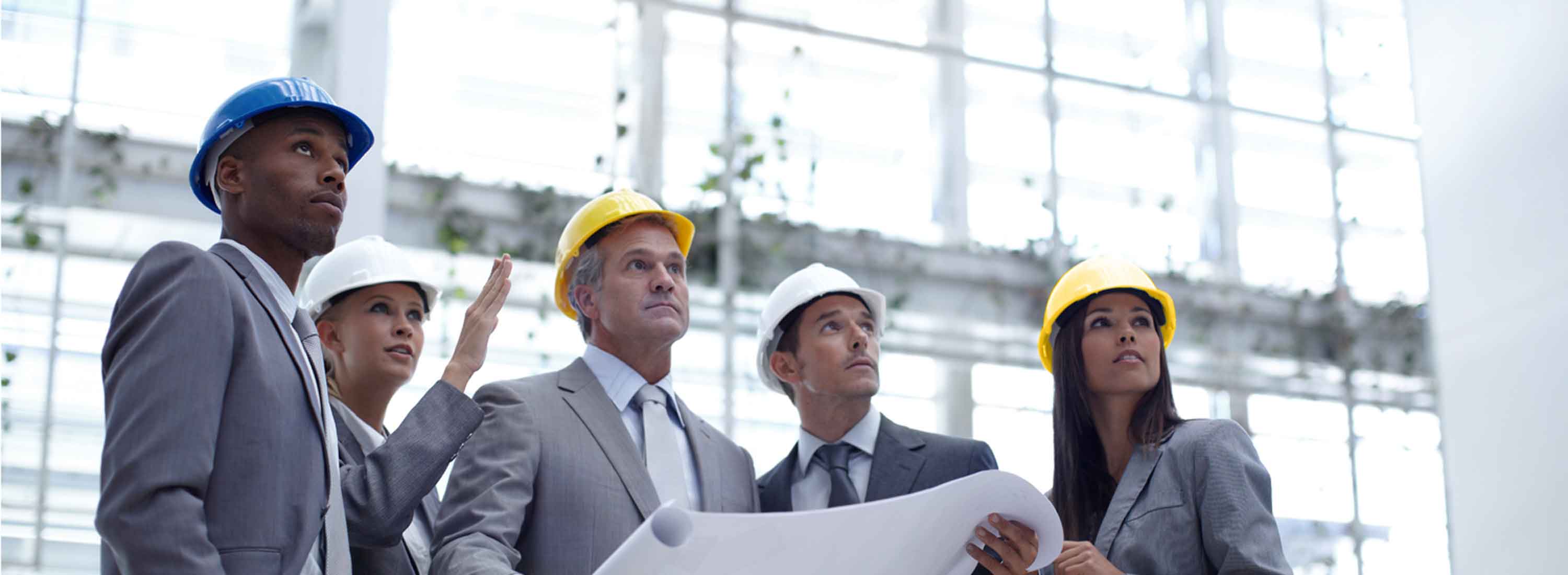
Persona |
Site Manager |
||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|
Fictional name |
Erlich Bochman |
||||||||||
Major Responsibilities |
Managing the construction sites that he is responsible for and monitor the work of site engineers |
||||||||||
Demographics |
45 year old, Site Manager , German and Married |
||||||||||
Goals and tasks |
As a manager, he is accountable for the successfull completion of building |
||||||||||
Environment |
Rather a tough environment, He has continous meeting with the site engineers |
||||||||||
Quote |
Reduce the mental pressure by using BIM app! |
Mockup of MoneyTracker application
Below is the prototype of Money Tracker application
Home screen :
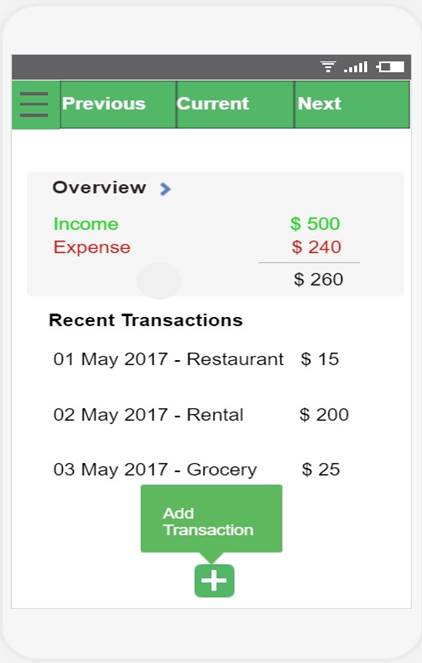
Add transaction screen :
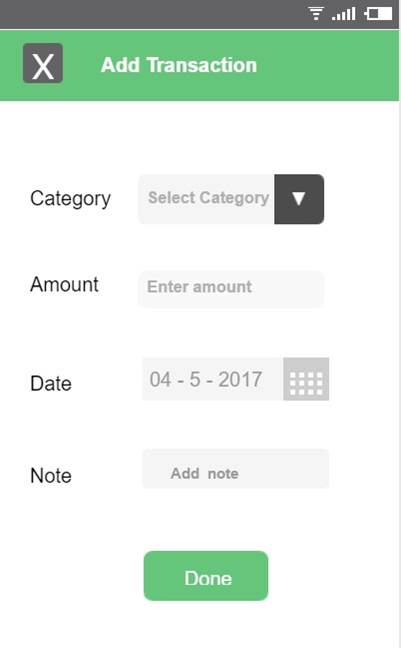
Story board of MoneyTracker application
1. Enabling login in settings window :
Shows the paper prototype for enabling of login action. From settings one can enable Login option and on entering the application for the next time, authentication and authorisation actions are performed.
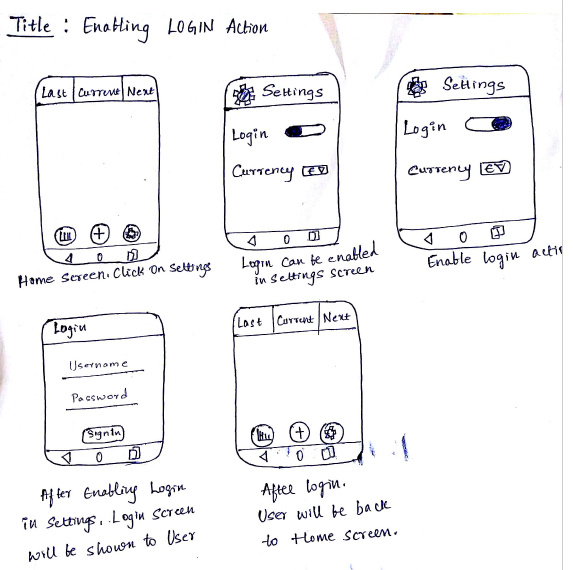
2. Adding transactions (Income or Expense) :
Shows the paper prototype for adding the Transaction. User can choose Income or Expense type and navigate to Add Transaction window where he/she will be asked to select subcategory of selected Income/Expense type, amount and Date. Once done filling and saving transaction, it's data will be updated in Homescreen.
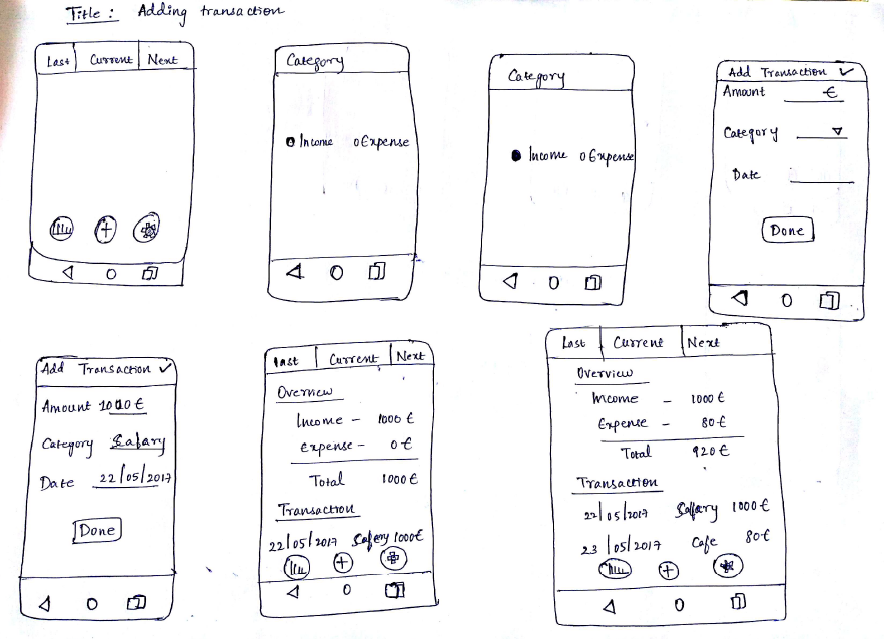
User interfaces
1. Login :
2. Add transaction :
3. Settings :
Summary of changes
After clarifying with the customer we made couple of changes. Below are the key changes to our requirements.
PIN enable and disable - By default, the app will not be password protected. If the user wants his app to be password protected, then he will have an option to enable PIN in settings. When he enables his PIN for the first time he will be redirected to a new screen where he can enter and save his user credentials. On subsequent login, he will have to enter his PIN to access the application.
Change currency option was moved to settings module. By default, the currency will be set to 'EURO', if the user wants to change the currency, then all the existing transactions would be deleted.